Ep.05: Readonly and Optional
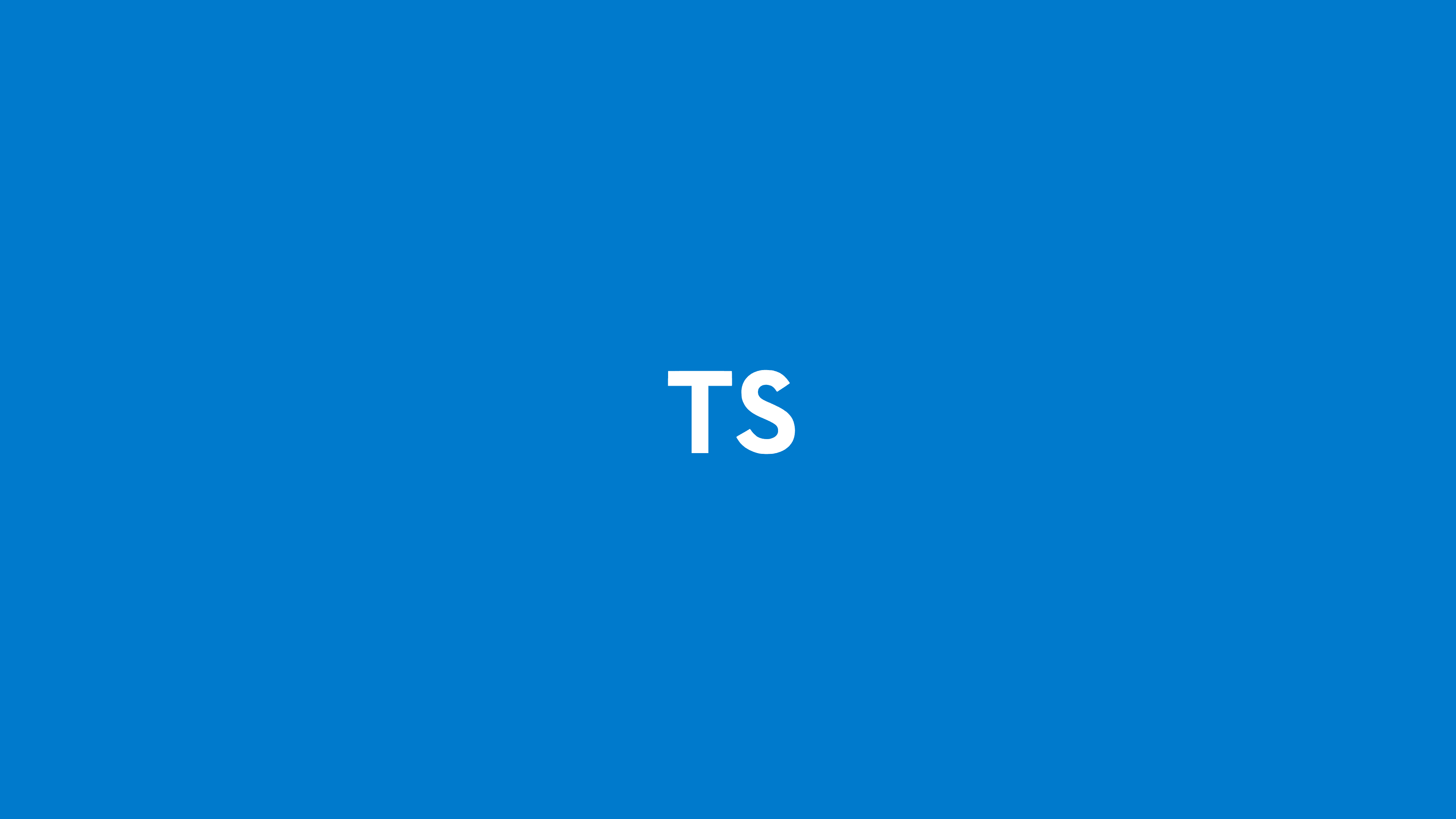
Readonly
is a keyword in typescript that makes any entity readonly or doesn't allow it to be manipulated.
To use it just write 'readonly' before the entity to make it readonly.
typescript
type User = { readonly _id: string; name: string; email: string }; let user1: User = { _id: "12f8", name: "John Doe", email: "john@xyz.com" } user1.email = "john.doe@abc.com"; user1._id = "42r3"; // ERROR: can't change readonly property
Optional (?)
allows us to make a property optional so that it don't throw an error if it's not available.
To make a property optional use "?" symbol after the name of the property and before the colon.
typescript
type User = { _id: string; name: string; email: string; location?: string }; let user2: User = { _id: "48t2", name: "Anthony", email: "anthony@xyz.com", // here location not passed but no error is thrown as it's optional. }